This article provides an overview for automation within Twitter, with a focus on automatic Tweets.
Twitter Automation Rules
First, you’ll want to familiarize yourself with Twitter’s automation rules. Of particular interest to this article is the Automatic Tweets section:
1. Posting automated Tweets
Automated Tweets that cross-post outside information: You may post automated Tweets based on sources of outside information — such as an RSS feed, weather data, etc. — as long as you are sufficiently authorized to publish such information.
Other automated Tweets (excluding mentions or replies): Provided you comply with all other rules, you may post automated Tweets for entertainment, informational, or novelty purposes. As a reminder, accounts posting duplicative, spammy, or otherwise prohibited content may be subject to suspension.
Twitter’s automation rules
Getting Started with Twitter Developer
You must apply for access to Twitter’s APIs and developer tools. The application consists of a handful of steps. After answering all questions and verifying your developer email, your application will be officially under review. Our application was approved within 12 hours in May 2021.
To get started, sign into the Twitter Developer Platform.
You will begin by storing your API keys. Be sure to keep these safe and secure. If all else fails, you can regenerate these keys, which will be required in a later step.
Note: there is a monthly cap of pulling 500,000 Tweets along with other limitations.
Follow this link to read the documentation on posting a tweet. Below are important points to consider.
While not rate limited by the API, a user is limited in the number of Tweets they can create at a time. If the number of updates posted by the user reaches the current allowed limit this method will return an HTTP 403 error.
You can only post 300 Tweets or Retweets during a 3 hour period.
Install Composer
Turn on SSH access, or use your preferred method to access a terminal. Enter the following commands to install Composer.
- php -r “copy(‘https://getcomposer.org/installer’, ‘composer-setup.php’);”
- php -r “if (hash_file(‘sha384’, ‘composer-setup.php’) === ‘756890a4488ce9024fc62c56153228907f1545c228516cbf63f885e036d37e9a59d27d63f46af1d4d07ee0f76181c7d3’) { echo ‘Installer verified’; } else { echo ‘Installer corrupt’; unlink(‘composer-setup.php’); } echo PHP_EOL;”
- php composer-setup.php
- php -r “unlink(‘composer-setup.php’);”
Install Twitter OAuth
For information about Twitter OAuth, visit this website or the Git Hub repository directly.
Within the terminal, enter:
- composer require abraham/twitteroauth
Enable Write Permissions and Regenerate Keys
Enabled read/write for your Twitter API app within the Twitter developer portal. Click your project’s app settings. Then find the App Permissions. Edit to allow at a minimum Read and Write.
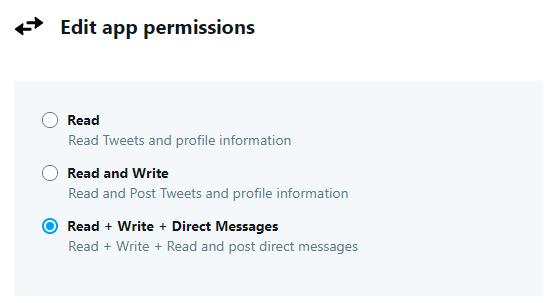
Regenerate your keys now before you start to use the Twitter OAuth library. Again, from the Twitter developer portal, where you clicked your project’s app settings, click the key icon instead. Regenerate all keys and store for later use.
CAUTION: you have to enable read, write access and regenerate your keys, including access token and access token secret, otherwise your original set of keys will not work to post tweets.
Create Script to Post Status
Example PHP script given below to post your first status. Note: be sure to replace your keys where indicated.
<?php
$consumer_key = <ENTER_KEY_HERE>;
$consumer_secret = <ENTER_KEY_HERE>;
$bearertoken = <ENTER_KEY_HERE>;
$access_token = <ENTER_KEY_HERE>;
$access_token_secret = <ENTER_KEY_HERE>;
require "../../../vendor/autoload.php";
use Abraham\TwitterOAuth\TwitterOAuth;
$connection = new TwitterOAuth($consumer_key, $consumer_secret, $access_token, $access_token_secret);
$status = $connection->post("statuses/update", ["status" => "hello world"]);
?>
There you have it. Now you can integrate automatic tweets into your application, system, or otherwise. You can also explore the countless other features the Twitter API affords you.